let과 const 그리고 변수
let으로 선언한 변수의 특징
- 변수의 값이 수시로 변경되어야 할때 사용한다.
- 명시한 타입 또는 타입 추론을 하여 타입이 결정되었을 때 해당 타입의 값으로만 값을 변경할 수 있다.
const로 선언한 변수의 특징
- 변수의 값이 변경되지 않을 때 사용한다.
- 생성할 때 초기값을 반드시 명시해야한다.
기본화면 코드 먼저 보여주겠다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
import React, { Component } from 'react';
import {View, Text, Button, Alert,StyleSheet} from 'react-native';
export default class App extends Component {
render(){
return(
<View style = {styles.container}>
<Text style = {styles.text}>근육 고양이</Text>
<Button title='실행' onPress={Template} />
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex:1,
justifyContent: 'center',
},
text: {
fontSize: 30,
textAlign: 'center'
}
})
|
위의 코드를 작성하면 근육 고양이를 얻을 수 있다.
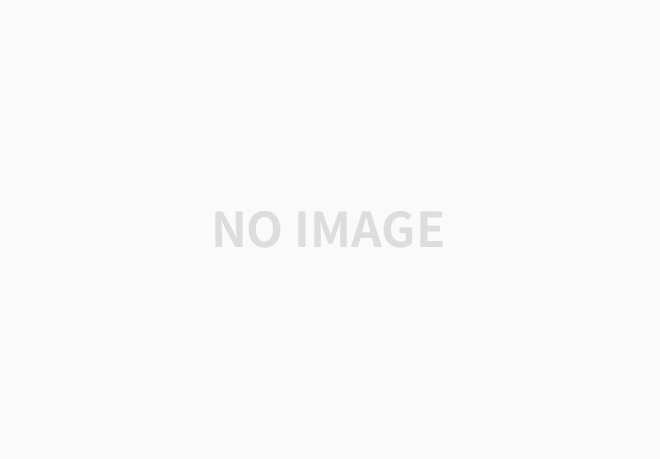
콘솔창을 통해서 보여줘야할 것도 있기 때문에 Button을 만들어 Button의 onPress에 함수를 할당하여 보여주기 위하여 기본화면을 만들었다.
let을 사용한 변수의 코드이다.
1
2
3
4
5
6
7
8
9
|
function KeywordTypeLet() {
let num:number = 1
let bool:boolean = true
let str:string = '근육 고양이'
num = false //Type 'false' is not assignable to type 'number'.
bool = '참' //Type '"참"' is not assignable to type 'boolean'.
str = 1 //Type '1' is not assignable to type 'string'.
}
|
코드를 작성하면 num, bool, str에 주석으로 표시한 오류들을 볼 수 있을 것이다. 처음 정해진 타입이 아닌 값으로는 값을 할당할 수 없다는 내용이다.
다음은 const를 사용한 변수의 코드이다.
1
2
3
4
5
6
7
8
9
|
function KeywordTypeConst() {
const num:number = 1
const bool:boolean = true
const str:string = '근육 고양이'
num = 2 //Cannot assign to 'num' because it is a constant.
bool = false //Cannot assign to 'bool' because it is a constant.
str = '콘스트 고양이' //Cannot assign to 'str' because it is a constant.
}
|
코드를 작성하면 마찬가지로 주석으로 표사한 오류들을 볼 수 있다. const로 선언된 변수들에는 다른 값을 할당할 수 없다는 내용이다. 여기서 알 수 있듯이 const로 선언한 변수들은 초기값으로만 초기화가 되고 다른 값으로 변할 수 없다.
any, undefined 타입
TypeScript에는 기본적으로 number, boolean, string, object, any, undefined 등의 타입들이 존재한다. any와 undefined는 무엇일까?
any 타입
모든 타입의 최상위 타입이다. 따라서 어떠한 타입의 값도 저장할 수 있는 변수 타입이다.
다음은 예제 코드이다.
1
2
3
4
5
6
|
function AnyType() {
let anyType: any
anyType = 1
anyType = true
anyType = '어떠한 고양이'
}
|
이처럼 any타입으로 선언된 변수는 어떠한 값도 저장할 수 있다.
undefined 타입
모든 타입의 최하위 타입이다. 따라서 아무런 타입의 값도 저정할 수 없는 변수 타입이다.
다음은 예제 코드이다.
1
2
3
4
5
6
|
function UndefinedType() {
let undefinedType: undefined
undefinedType = 2 //Type '2' is not assignable to type 'undefined'.
undefinedType = false //Type 'false' is not assignable to type 'undefined'.
undefinedType = '아무런 고양이' //Type '"아무런 고양이"' is not assignable to type 'undefined'.
}
|
이처럼 undefined타입으로 선언된 변수는 아무런 타입의 값도 저장할 수 없다.
여기까지 TypeScript 변수와 타입에 대한 글이다. TypeScript에 대한 정보 없이 리액트 네이티브를 공부하니까 내가 타입스크립트를 제대로 쓰고는 있는 것인지에 대한 의문이 들어 기초부터 차근차근 올리려고 한다.
'Project > 근육고양이' 카테고리의 다른 글
[근육고양이] TypeScript 심화 함수 (0) | 2020.04.28 |
---|---|
[근육고양이] TypeScript 함수 (0) | 2020.04.27 |
[근육고양이] TypeScript 클래스 (0) | 2020.04.26 |
[근육고양이] TypeScript 객체와 인터페이스 (0) | 2020.04.25 |
[근육고양이] 기획 (0) | 2020.04.23 |